How to use MQTT in PHP
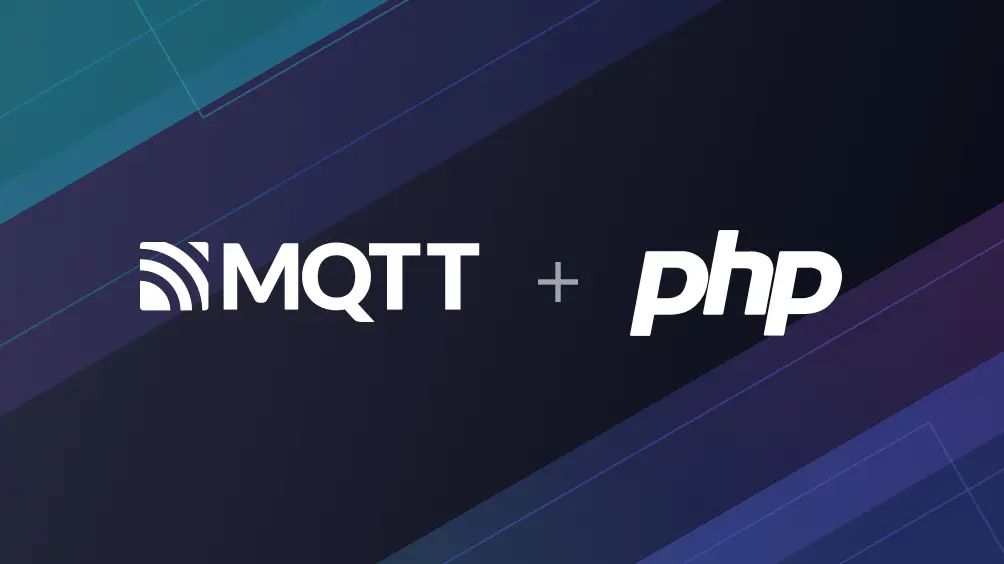
PHP is a widely-used open source multi-purpose scripting language, which can be embedded in HTML and is especially suitable for Web development.
This article mainly introduces how to use the php-mqtt/client
client library in PHP projects to implement the functions of connection, subscription, unsubscribing, message receiving and sending between MQTT client and MQTT broker.
MQTT client library selection
This article chooses the client library php-mqtt/client
, which has the highest downloads on composer. For more PHP-MQTT client libraries, please view in Packagist-Search MQTT.
For more documentation about php-mqtt/client, please refer to Packagist php-mqtt/client.
MQTT communication belongs to a network communication scenario outside the HTTP system. Due to the limitation of PHP characteristics, using the extensions for network communication such as Swoole/Workerman in the PHP system can bring a better experience. Its use will not be repeated in this article. The relevant MQTT client libraries are as follows:
- workerman/mqtt:Asynchronous MQTT client for PHP based on workerman.
- simps/mqtt:MQTT protocol Analysis and Coroutine Client for PHP.
Project initialization
Confirm the PHP version
This project uses 7.4.21 for development and testing. Readers can confirm the PHP version with the following command.
php --version
PHP 7.4.21 (cli) (built: Jul 12 2021 11:52:30) ( NTS )
Copyright (c) The PHP Group
Zend Engine v3.4.0, Copyright (c) Zend Technologies
with Zend OPcache v7.4.21, Copyright (c), by Zend Technologies
Use Composer to install php-mqtt/client
Composer is a dependency management tool for PHP, which can manage all the dependencies your PHP project needs.
composer require php-mqtt/client
PHP MQTT usage
Connect to the MQTT broker
This article will use the free public MQTT broker provided by EMQX, which is created on EMQX's MQTT Cloud Service. The server access information is as follows:
- Broker: broker.emqx.io
- TCP Port: 1883
- SSL/TLS Port: 8883
Import composer autoload file and php-mqtt/client
require('vendor/autoload.php');
use \PhpMqtt\Client\MqttClient;
Set MQTT Broker connection parameters
Set the MQTT Broker connection address, port and topic. At the same time, we call the PHP rand
function to generate the MQTT client id randomly.
$server = 'broker.emqx.io';
$port = 1883;
$clientId = rand(5, 15);
$username = 'emqx_user';
$password = 'public';
$clean_session = false;
$mqtt_version = MqttClient::MQTT_3_1_1;
Write the MQTT connection function
Use the above parameters to connect, and set the connection parameters through ConnectionSettings
, such as:
$connectionSettings = (new ConnectionSettings)
->setUsername($username)
->setPassword($password)
->setKeepAliveInterval(60)
->setLastWillTopic('emqx/test/last-will')
->setLastWillMessage('client disconnect')
->setLastWillQualityOfService(1);
Subscribe
Program to subscribe to the topic of emqx/test
, and configure a callback function for the subscription to process the received message. Here we print out the topic and message obtained from the subscription:
$mqtt->subscribe('emqx/test', function ($topic, $message) {
printf("Received message on topic [%s]: %s\n", $topic, $message);
}, 0);
Publish
Construct a payload and call the publish
function to publish messages to the emqx/test
topic. After publishing, the client needs to enter the polling status to process the incoming messages and the retransmission queue:
for ($i = 0; $i< 10; $i++) {
$payload = array(
'protocol' => 'tcp',
'date' => date('Y-m-d H:i:s'),
'url' => 'https://github.com/emqx/MQTT-Client-Examples'
);
$mqtt->publish(
// topic
'emqx/test',
// payload
json_encode($payload),
// qos
0,
// retain
true
);
printf("msg $i send\n");
sleep(1);
}
// The client loop to process incoming messages and retransmission queues
$mqtt->loop(true);
Complete code
Server connection, message publishing and receiving code.
<?php
require('vendor/autoload.php');
use \PhpMqtt\Client\MqttClient;
use \PhpMqtt\Client\ConnectionSettings;
$server = 'broker.emqx.io';
$port = 1883;
$clientId = rand(5, 15);
$username = 'emqx_user';
$password = 'public';
$clean_session = false;
$mqtt_version = MqttClient::MQTT_3_1_1;
$connectionSettings = (new ConnectionSettings)
->setUsername($username)
->setPassword($password)
->setKeepAliveInterval(60)
->setLastWillTopic('emqx/test/last-will')
->setLastWillMessage('client disconnect')
->setLastWillQualityOfService(1);
$mqtt = new MqttClient($server, $port, $clientId, $mqtt_version);
$mqtt->connect($connectionSettings, $clean_session);
printf("client connected\n");
$mqtt->subscribe('emqx/test', function ($topic, $message) {
printf("Received message on topic [%s]: %s\n", $topic, $message);
}, 0);
for ($i = 0; $i< 10; $i++) {
$payload = array(
'protocol' => 'tcp',
'date' => date('Y-m-d H:i:s'),
'url' => 'https://github.com/emqx/MQTT-Client-Examples'
);
$mqtt->publish(
// topic
'emqx/test',
// payload
json_encode($payload),
// qos
0,
// retain
true
);
printf("msg $i send\n");
sleep(1);
}
$mqtt->loop(true);
Test
After running the MQTT message publishing code, we will see that the client has successfully connected, and the messages have been published one by one and received successfully:
php pubsub_tcp.php
Summary
So far, we have used the php-mqtt/client to connect to the free public MQTT broker, and implemented the connection, message publishing and subscription between the test client and the MQTT server.
Next, you can check out The Easy-to-understand Guide to MQTT Protocol series of articles provided by EMQ to learn about MQTT protocol features, explore more advanced applications of MQTT, and get started with MQTT application and service development.